Arduino Serial Port Line Ending Characters
Arduino Serial Port write function send all the character in the string specified.
write() function to send a string
Serial.write(“Hello World!”);
Note: This code simply send the 12 bytes one by one to send the complete string over serial port.
Many times we need to send some additional character after sending the specified string. Suppose you are interfacing the Arduino with the devices like GPS, GSM etc.
In these cases you use serial port to send command to these devices, and these devices requires command ending character like carriage return CR and line feed LF.
Carriage return CR and line feed LF are not alpha-numeric characters, it is really tricky to send with Arduino serial port.
Both carriage return CR and line feed LF are defined in the ASCII code and it can be sent with its decimal values.
Both carriage return CR and line feed LF are known as control characters, they don’t have any visual meaning.
Caution: The carriage return CR ASCII decimal code is 13 and line feed LF or new line ASCII decimal code is 10.
How Arduino Serial Port Can Send or Write Carriage Return CR Character
To send the CR carriage return character you can use write() function with escape character or with its decimal value.
write() function to send a string with CR in the end
Serial.write(“Hello World!\r”);
write() function to send a CR with its decimal value
Serial.write(13);
Note: “\r” is a special character specify the CR. “\” is known as escape character, which used to specify the some special character in the string. Many printable and and non-printable characters are need to be specified with the help of escape symbol.
How Arduino Serial Port Can Write Line Feed LF and New Line Character
To send the LF line feed or new line character you can use write() function with escape character or with its decimal value.
write() function to send a string with line feed or new line in the end
Serial.write(“Hello World!\n”);
write() function to send a LF with its decimal value
Serial.write(10);
Note: “\n” is a special character specify the LF.
write() function to send a string with both carriage return and line feed in the end
Serial.write(“Hello World!\r\n”);
Arduino Serial Print New Line Character
In place of write() function you can use print function as well to send the CR and LF.
print() function to example code to send carriage return and line feed
Serial.print(“Hello World!\r\n”);
Caution: You can send the special character with its octal and hexadecimal values as well in the string. To send LF with hexadecimal value you have to use syntax \x0a , and to send CR the syntax is \x0d.
How Arduino Serial Monitor Can Send Carriage Return CR and Line Feed LF Character
The serial monitor application is build-in in the Arduino IDE. In the serial monitor tool, when Arduino send the new line, the next printable character will be displayed in the new fresh line.
The serial monitor application is also able to send the CR and LF special characters.
In the figure 1 shows the option for line ending character (near baud rate option).
In this option there are four choices-
- No Line Ending – It means no additional character sent as line ending.
- Carriage Return – Send \r in the end, after the specified string (CR).
- Newline – Send \n after the specified string (LF).
- Both NL & CR – Send both \r and then \n in the end.
Arduino Serial Monitor Test with Line Ending Character
To test the CR and LF character we create a small loop-back code, which actually send back, everything it receives.
In this code we have used Serial.println() function to send back the received data.
Example 1 – Serial.println function to send the ASCII decimal value of the character.
/* Serial port test with serial monitor tool Author: Nilesh Chaurasia https://elextutorial.com */ void setup() { Serial.begin(9600); // Set the baud rate to 9600 } void loop() { if(Serial.available()) // Check if received { int data1 = Serial.read(); // Read if received Serial.println(data1); // Send back to PC/Laptop } }
This is a simple loop back program but with print() function, which actually send the integer decimal value of the character received.

In the first test (No line ending) we type “abc” and click on send button. The serial monitor send only three characters and receive back (97,98 and 99) which are actually decimal equivalent of the ASCII codes of a,b and c.
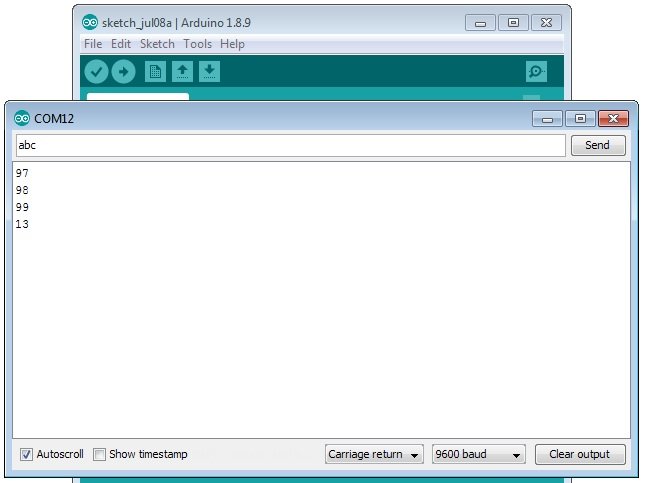
In the second test (Carriage Return) we again type “abc” and click on send button. The serial monitor send now four characters and receive back (97,98,99 and 13). 13 is actually decimal equivalent of the ASCII code of CR.

In the third test (Newline) we again type “abc” and click on send button. The serial monitor send now four characters and receive back (97,98,99 and 10). 10 is actually decimal equivalent of the ASCII code of Newline (LF).
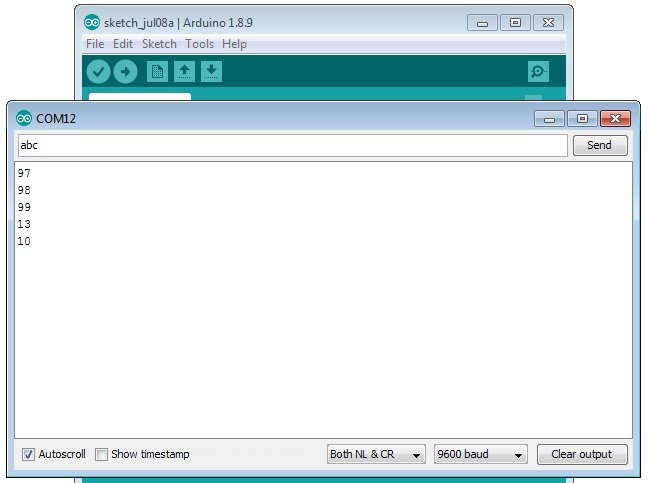
In the fourth test (Both NL & CR) we again type “abc” and click on send button. The serial monitor send now five characters and receive back (97,98,99,13 and 10). 13 and 10 are actually decimal equivalent of the ASCII code of NL and CR.
Wow that was unusual. I just wrote an really long comment but after I clicked submit my comment didn’t show up. Grrrr… well I’m not writing all that over again. Regardless, just wanted to say fantastic blog!
Hi would you mind letting me know which web host you’re utilizing? I’ve loaded your blog in 3 different web browsers and I must say this blog loads a lot quicker then most. Can you suggest a good hosting provider at a honest price? Cheers, I appreciate it!
I really like looking through your post and I think this website got some really useful stuff on it!
I was recommended this website by my cousin. I am not sure whether this post is written by him as no one else know such detailed about my trouble. You’re wonderful! Thanks!