PHP while Loop Statement
PHP while Loop is used to execute the part of code multiple times. This Loop is also working similarly to the for loop but has a little bit change in the syntax.
All the three statements are broke down into 3 part. Firstly we initialize the variable than give the condition and then increment/decrement statement.
while Loop Syntax
<!DOCTYPE html> <html> <head> </head> <body> <?php initialization; while(condition) { code to be executed; increment/decrement; } ?> </body> </html>
Example 1 – while Loop Example code to print series of numbers
<!DOCTYPE html> <html> <head> </head> <body> <?php $x = 1; while($x<=5) { echo $x . "<br />"; $x++; } ?> </body> </html>
In the above code, we describe how you can use while Loop and here we print the counting from 1 to 5.
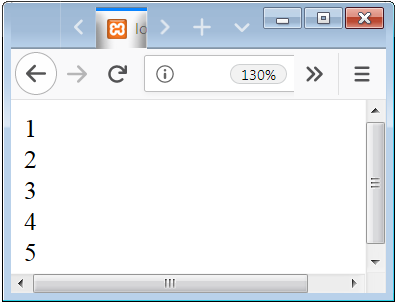
Print the Table of 2 using while Loop
The given below code is used to print the table of two with while Loop
Example 2 – Print the table of 2 using while Loop Example
<!DOCTYPE html> <html> <head> </head> <body> <?php $x = 1; while($x<=10) { echo "2 X " . $x . " = " . $x * 2 . "<br />"; $x++; } ?> </body> </html>
In the above code, we describe how you can use while Loop and here we print the table of 2.

PHP while Loop Break and Continue Statement Syntax and Example
Break and Continue both is used to for the different purpose such Break is used to stop the loop and where Continue is used to skip the statement.
Example 3 – while Loop Break Syntax Example to exit from the loop in certain condition
<!DOCTYPE html> <html> <head> </head> <body> <?php $x = 1; while($x<=10) { echo "2 X " . $x . " = " . $x * 2 . "<br />"; if($x==5) { break; } $x++; } ?> </body> </html>
In the above code, we describe how you can use while Loop and here we print the table of 2 but we break the loop when the value of variable $x will be 5 then the execution we will not do and the execution will end.
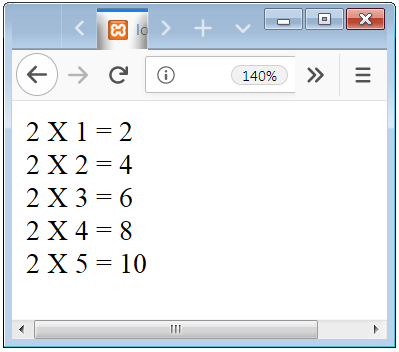
Example 4 – while Loop Continue Example Code to skip certain condition
<!DOCTYPE html> <html> <head> </head> <body> <?php $x = 0; while($x<10) { $x++; if($x==7) { continue; } echo "2 X " . $x . " = " . $x * 2 . "<br />"; } ?> </body> </html>
In the above code, we describe how you can use while Loop and here we print the table of 2 but we Continue the loop when the value of variable $x will be 7 then the execution will be skipped once.
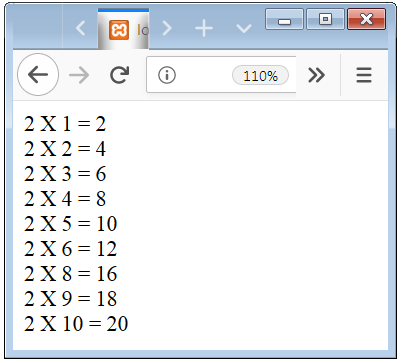
Note: You can use break and continue in do while loop also.
PHP do while Loop
PHP do while loop is also used to execute a part of the code for specified times. This loop is also similar to for and while but have different syntax and working procedure.
do….while Loop Syntax
<!DOCTYPE html> <html> <head> </head> <body> <?php initialization; do { code to be executed; increment/decrement; }while(condition) ?> </body> </html>
Example 5 – do….while Loop Example code to print series of numbers
<!DOCTYPE html> <html> <head> </head> <body> <?php $x = 0; do { $x++; echo $x . "<br />"; }while($x<=5) ?> </body> </html>
In the above code, we describe how you can use do while Loop and here we print the counting from 1 to 5. the loop will be stopped when the value of $x will less than or equal to 5.
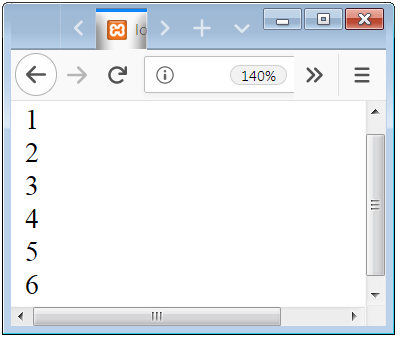
Difference between while & do…while Loop
The major difference between the while loop and do….while loop is that the while loop checks the condition first and then execute the code where in do…while loop firstly executes the code then check the condition. It means that the do…while loop executes at least once even if the condition is false.
Example 6 – Demonstrate the difference between them
<!DOCTYPE html> <html> <head> </head> <body> <?php $x = 7; do { $x++; echo $x . "<br />"; }while($x<=5) ?> </body> </html>
In the above code, we can use do while Loop and the value of the variable $x is 7 but the condition is not valid to that variable. But the execution of the loop will start and execute the code once. In the above code, we can use do while Loop and the value of the variable $x is 7 but the condition is not valid to that variable. But the execution of the loop will start and execute the code once.
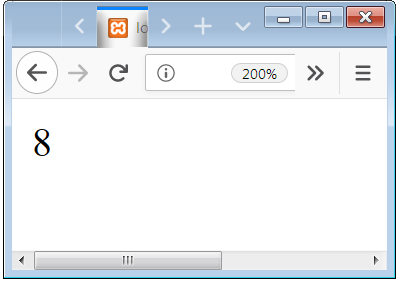