PHP Array Functions
Array Function is used to perform the basic task in an array for example count(), array sum(), array search and many more.
List of popular pre-defined Array Functions
Fuctions | Description |
---|---|
array() | To create an array. |
count() | To known the number of elements inside an array. |
array_sum() | To get the addition of values of array. |
array_search() | To find a particular value of array and get the key. |
array_reverse() | To get the reverse order of an array. |
array_replace() | To replace the value of first array from the another one array. |
PHP Array count
The count() function is used to get the number of elements are available in an array.
Example 1 – count() Function Example Code
<!DOCTYPE html> <html> <head> </head> <body> <?php $marks = array ( array("Peter","Maths",89), array("Peter","Physics",66), array("Juliet","Maths",99), array("Juliet","Physics",74) ); echo "The number of element in array " . count($marks); ?> </body> </html>
The above example has a multidimensional array and we use count() function to know the number elements in an array.
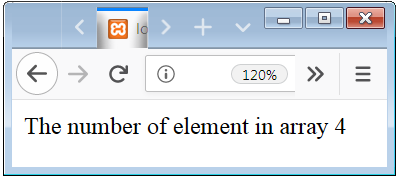
PHP Array Add Function
The array sum() function is used to get the addition of the values of an array.
Example 2 – array_sum() Function Example
<!DOCTYPE html> <html> <head> </head> <body> <?php $sum = array(5,10,15,20); echo "The sum of the values of an array " . array_sum($sum); ?> </body> </html>

PHP Array Search Key Function
The array_search() function is used to find a particular value in an array and get the key of that particular value.
Example 3 – array_search() Function Example
<!DOCTYPE html> <html> <head> </head> <body> <?php $marks = array("Maths" => 98, "Physics" => 54, "Chemistry" => 56, "Maths" => 67); echo array_search(67,$marks); ?> </body> </html>

PHP Array Reverse
The array_reverse() function is used to get an array in the reverse order.
Example 4 – array_reverse() Function Example
<!DOCTYPE html> <html> <head> </head> <body> <?php $marks = array("Maths" => 98, "Physics" => 54, "Chemistry" => 56); print_r ($marks); echo "<br />"; print_r (array_reverse($marks)); ?> </body> </html>

PHP Array Replace Function
The array_replace() function is used to replace the value of the first array to the value of another array.
Example 5 – array_replace() Function Example
<!DOCTYPE html> <html> <head> </head> <body> <?php $marks1 = array("Maths", "Physics", "Chemistry", "English"); $marks2 = array("Biology", "Zology", "Hindi"); print_r ($marks1); echo "<br />"; print_r ($marks2); echo "<br />"; print_r (array_replace($marks1,$marks2)); ?> </body> </html>
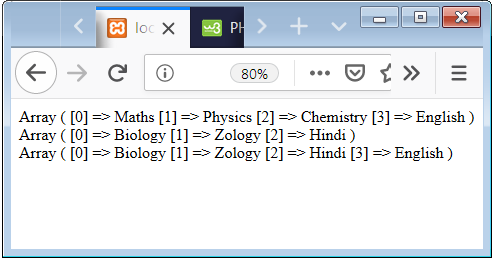