CSS Media Query
Media Query is used to changing the CSS Properties Values according to the width of the screen. CSS Properties like font-size, color, width and other.
Example 1 – CSS Media Query Example for responsive design
<!DOCTYPE html> <html> <head> <style> .col { float:left; width:50%; background-color:yellow; border:5px solid white; box-sizing:border-box; height:150px; } @media screen and (max-width: 800px) { .col { width:50%; } } @media screen and (max-width: 450px) { .col { width:100%; } } </style> </head> <body> <div class="two-column"> <div class="col"> <p></p> </div> <div class="col"> <p></p> </div> </div> </body> </html>
In the above examples, we have a two column design. Both column show as inline when the width of the screen is above the breakpoint(450px).
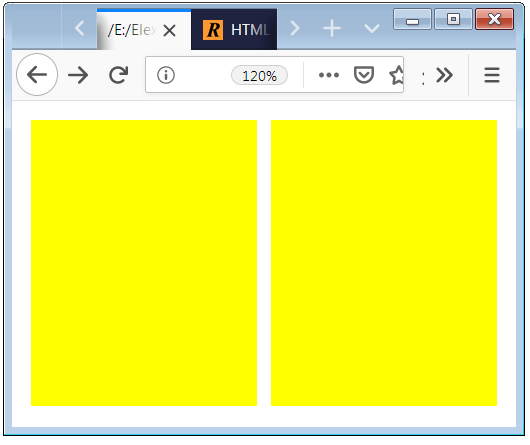
When you decrease the width of the screen which is below the breakpoint(450px) then one column will be shifted downwards. Which is shown in the below image.

What is Media Query Breakpoints?
Breakpoints is a pixel value at which you can change the different CSS properties value.
CSS Media Screen or Print
If you want to change the font size to the respect of screen size width. Below example is used to explain how you can change the font-size to the respect of the screen size.
Example 2 – Media as Screen Code Example
<!DOCTYPE html> <html> <head> <style> .col { float:left; width:50%; height:150px; border: 6px solid white; box-sizing: border-box; background-color: orange; } p { font-size: 35px; margin: 0; padding:0; } @media screen and (max-width: 900px) { .col { width:50%; } } @media screen and (max-width: 550px) { .col { width:100%; } p { font-size:14px; } } </style> </head> <body> <div> <div class="col"> <p>Lorem ipsum dolor sit amet consectetur adipiscing. Non tellus orci ac auctor augue mauris augue.</p> </div> <div class="col"> <p>Lorem ipsum cepteur sint occaecat cupidatat non proident, sunt in culpa qui officia deserunt.</p> </div> </div> <body> </html>
In the above examples, we have a two column design. Both column show as inline when the width of screen is above the breakpoint(550px).

When the width of the screen is below the breakpoint(550px) then one column will be shifted downwards and the font size will be changed from 35px to 14px.

Making Some content Invisible at Specific Width
If you want to some part invisible at a particular width of the screen. Below Code shows how you make content invisible.
Example 3 – Making Some content at Specific Width Example
<html> <head> <style> .col { float:left; width:50%; height:150px; background-color:orange; border: 2px solid white; box-sizing: border-box; } div img { width: 100%; } @media screen and (max-width: 900px) { .col { width:50%; } } @media screen and (max-width: 450px) { .col { width:100%; } div.image { display:none; } } </style> </head> <body> <div> <div class="image"> <img src="tulip.jpg" /> </div> <div class="col"> <p></p> </div> <div class="col"> <p></p> </div> </div> <body> </html>
In the above examples, we have a two column design with a image of tulip flower. Both column show as inline when the width of screen is above the breakpoint(450px).

When the width of the screen is below the breakpoint(450px) then one column will be shifted downwards and the image will not be visible(or invisible).

Responsive Layout Design
The given code shows how you can create a Two-Column responsive Layout with Header and Footer.
Example 4 – Responsive Layout Design Example
<!DOCTYPE html> <html> <head> <style> .container { } .header { background-color:yellow; border: 1px solid yellow; } .header-inner { background-color:white; height:30px; margin:10px; } .left-sidebar { float:left; width:40%; height:70px; background-color:orange; } .left-sidebar-inner { background-color:white; height:50px; margin:10px; } .right-sidebar { float:right; width:60%; height:70px; background-color:green; } .right-sidebar-inner { background-color:white; height:50px; margin:10px; } .cleaner { clear:both; } .footer { height:50px; background-color:brown; padding:1px; } .footer-inner { background-color:white; height:30px; margin:9px; } @media screen and (max-width: 450px) { .right-sidebar { width:100%; } .left-sidebar { width:100%; } } </style> </head> <body> <div class="container"> <div class="header"> <div class="header-inner"> </div> </div> <div class="left-sidebar"> <div class="left-sidebar-inner"> </div> </div> <div class="right-sidebar"> <div class="right-sidebar-inner"> </div> </div> <div class="cleaner"> </div> <div class="footer"> <div class="footer-inner"> </div> </div> </div> </body> </html>
In the above examples, we have a div layout. Below figure shows the design of layout at full screen.
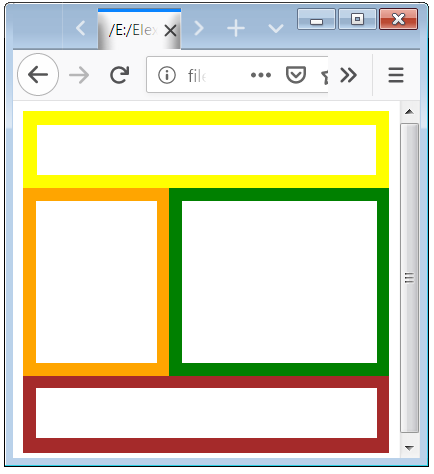
When the width of the screen is below the breakpoint(450px) then all columns will be arranged or shifted downwards.
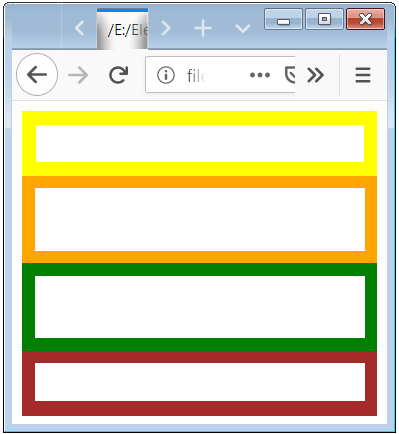